Auth manager¶
Auth (for authentication/authorization) manager is the component in Airflow to handle user authentication and user authorization. They have a common API and are “pluggable”, meaning you can swap auth managers based on your installation needs.
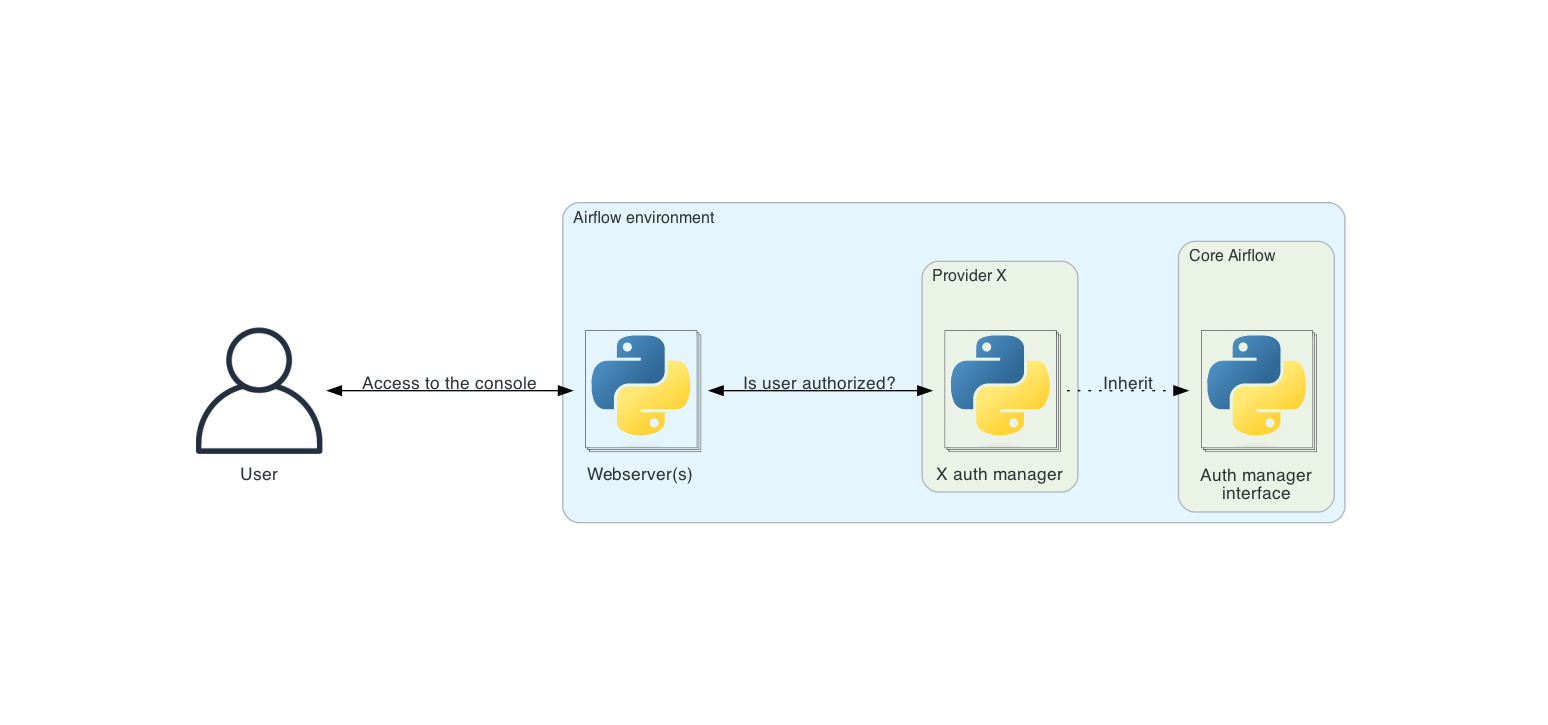
Airflow can only have one auth manager configured at a time; this is set by the auth_manager
option in the
[core]
section of the configuration file.
Note
For more information on Airflow’s configuration, see Setting Configuration Options.
If you want to check which auth manager is currently set, you can use the
airflow config get-value core auth_manager
command:
$ airflow config get-value core auth_manager
airflow.providers.fab.auth_manager.fab_auth_manager.FabAuthManager
Why pluggable auth managers?¶
Airflow is used by a lot of different users with a lot of different configurations. Some Airflow environment might be used by only one user and some might be used by thousand of users. An Airflow environment with only one (or very few) users does not need the same user management as an environment used by thousand of them.
This is why the whole user management (user authentication and user authorization) is packaged in one component called auth manager. So that it is easy to plug-and-play an auth manager that suits your specific needs.
By default, Airflow comes with the Flask AppBuilder (FAB) auth manager.
Note
Switching to a different auth manager is a heavy operation and should be considered as such. It will impact users of the environment. The sign-in and sign-off experience will very likely change and disturb them if they are not advised. Plus, all current users and permissions will have to be copied over from the previous auth manager to the next.
Writing your own auth manager¶
All Airflow auth managers implement a common interface so that they are pluggable and any auth manager has access to all abilities and integrations within Airflow. This interface is used across Airflow to perform all user authentication and user authorization related operation.
The public interface is BaseAuthManager
.
You can look through the code for the most detailed and up to date interface, but some important highlights are
outlined below.
Note
For more information about Airflow’s public interface see Public Interface of Airflow.
Some reasons you may want to write a custom auth manager include:
An auth manager does not exist which fits your specific use case, such as a specific tool or service for user management.
You’d like to use an auth manager that leverages an identity provider from your preferred cloud provider.
You have a private user management tool that is only available to you or your organization.
Optional methods recommended to override for optimization¶
The following methods aren’t required to override to have a functional Airflow auth manager. However, it is recommended to override these to make your auth manager faster (and potentially less costly):
batch_is_authorized_dag
: Batch version ofis_authorized_dag
. If not overridden, it will callis_authorized_dag
for every single item.batch_is_authorized_connection
: Batch version ofis_authorized_connection
. If not overridden, it will callis_authorized_connection
for every single item.batch_is_authorized_pool
: Batch version ofis_authorized_pool
. If not overridden, it will callis_authorized_pool
for every single item.batch_is_authorized_variable
: Batch version ofis_authorized_variable
. If not overridden, it will callis_authorized_variable
for every single item.get_permitted_dag_ids
: Return the list of DAG IDs the user has access to. If not overridden, it will callis_authorized_dag
for every single DAG available in the environment.filter_permitted_menu_items
: Return the menu items the user has access to. If not overridden, it will callhas_access
inAirflowSecurityManagerV2
for every single menu item.
CLI¶
Auth managers may vend CLI commands which will be included in the airflow
command line tool by implementing the get_cli_commands
method. The commands can be used to setup required resources. Commands are only vended for the currently configured auth manager. A pseudo-code example of implementing CLI command vending from an auth manager can be seen below:
@staticmethod
def get_cli_commands() -> list[CLICommand]:
sub_commands = [
ActionCommand(
name="command_name",
help="Description of what this specific command does",
func=lazy_load_command("path.to.python.function.for.command"),
args=(),
),
]
return [
GroupCommand(
name="my_cool_auth_manager",
help="Description of what this group of commands do",
subcommands=sub_commands,
),
]
Note
Currently there are no strict rules in place for the Airflow command namespace. It is up to developers to use names for their CLI commands that are sufficiently unique so as to not cause conflicts with other Airflow components.
Note
When creating a new auth manager, or updating any existing auth manager, be sure to not import or execute any expensive operations/code at the module level. Auth manager classes are imported in several places and if they are slow to import this will negatively impact the performance of your Airflow environment, especially for CLI commands.
Rest API¶
Auth managers may vend Rest API endpoints which will be included in the REST API Reference by implementing the get_api_endpoints
method. The endpoints can be used to manage resources such as users, groups, roles (if any) handled by your auth manager. Endpoints are only vended for the currently configured auth manager.
Next Steps¶
Once you have created a new auth manager class implementing the BaseAuthManager
interface, you can configure Airflow to use it by setting the core.auth_manager
configuration value to the module path of your auth manager:
[core]
auth_manager = my_company.auth_managers.MyCustomAuthManager
Note
For more information on Airflow’s configuration, see Setting Configuration Options and for more information on managing Python modules in Airflow see Modules Management.